Overview
Bank Address Book (BankAB) is a business process management and workflow application platform, whereby its users are all the employees in a banking environment. This platform supports the basic daily routine of each employee such as:
-
Checking working schedule
-
Applying for leave application
-
Checking in and out during working hours
The managers and administrators will have higher priority access level of BankAB such as changing the priority level of the employee and approve or reject leave requests.
Summary of contributions
-
Major enhancement 1: implemented user account with corresponding APIs and commands.
-
What it does: This allows all users to have a unique account to login and perform functions that are essential for work purpose.
-
Justification: This feature is a core component of the product as certain functions (such as leave application), require user authentication.
-
Highlights: This enhancement is challenging as we need to modify a major portion of existing source codes and test codes, owing to the fact that the newly added NRIC parameter is unique and other existing fields, which are initially unique, are now not. In addition, many functions that are developed by my team is dependent on this feature. This results in the pressure on me to deliver this feature at the shortest time possible.
-
-
Major enhancement 2: implemented priority access level with corresponding APIs and commands.
-
What it does: All user accounts will have a priority level, which will allow/restrict them to functions that require an elevated user to do so.
-
Justification: This feature is also a core component and complements the implementation of user accounts, as some features (e.g.: Approving of leaves), require someone of a senior rank to do so. This will prevent users from potentially abusing company resources and product functions.
-
-
Code contributed: Code Dashboard
-
Minor enhancement: added a reset command to reset the Bank Address Book to the original state at first start
-
Other contributions:
-
Project management:
-
Set up the organization and project repo on Github
-
Managed vetting through and approving individual pull requests
-
Deployed website for our project: Website
-
-
Enhancements to existing features:
-
Added a field that shows the user who is logged in at the status footer of the UI
-
Made significant changes to priority level ranking following the feedback received from the first practical exam: #134
-
-
Documentation:
-
Re-arranged summary of commands in the user guide to make them more organized: #89
-
Added appendices A and B in the user guide that summarizes the prefixes and corresponding data types, and also various priority levels and its corresponding privileges: #60, #89
-
Updated documentations as the project progresses: #60, #68, #89, #96, #136, #144
-
-
Community:
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Personal User Account
Login to the application: login
Logs in to the application using a registered NRIC and password.
Format: login ic/NRIC pwd/PASSWORD
Example: login ic/S1234567E pwd/Password
A successful login will show the NRIC and name which you have used to login, followed by the schedule of the day (if any).

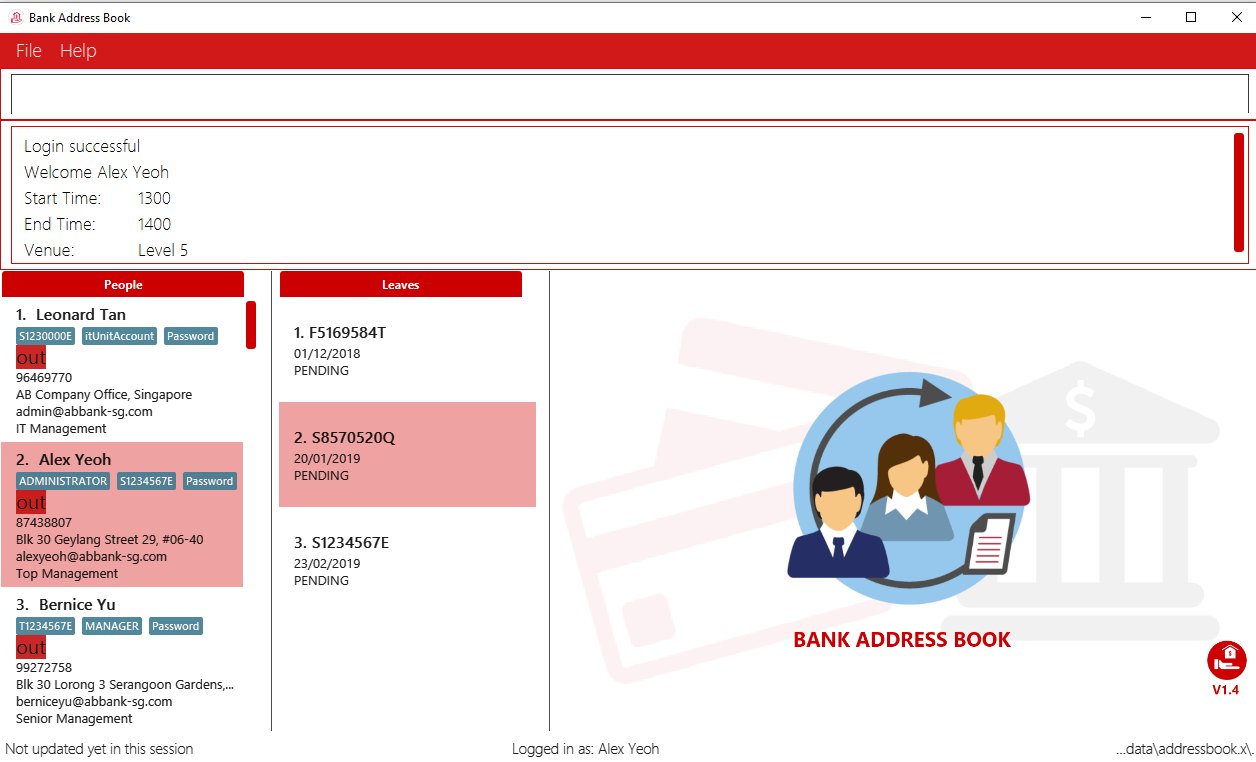
Checks the login status of the application: checkloginstatus
Prints whether this application is logged in.
Also prints the logged in NRIC if logged in.
Logout of the application: logout
Logs out of the application, allowing for other users to log in again.
Change priority level of employee: setplvl
|
Sets the priority level of an employee at the specified index.
Format: setplvl INDEX plvl/PRIORITY_LEVEL
Example: setplvl 1 plvl/0
-
A successful change of priority level should show the following message:
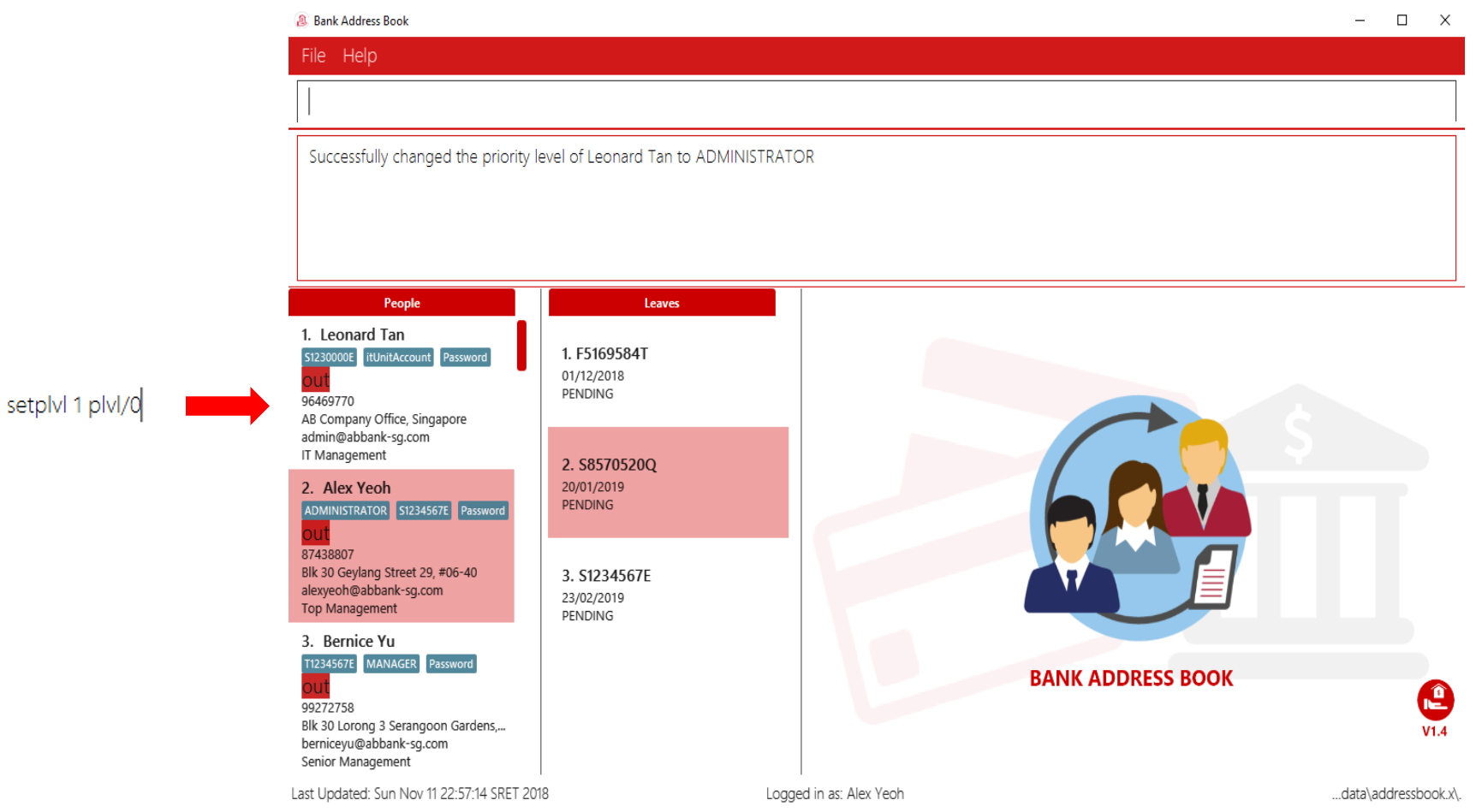
-
Users of insufficient priority level (below administrator priority level) will get the following error message when attempting to execute this command:
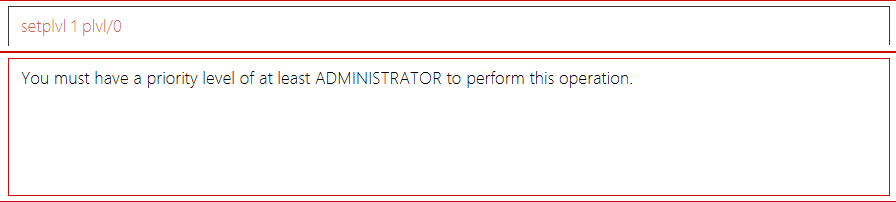
-
Users (with sufficient priority level), that attempts to change their own priority level will not be allowed to do so:
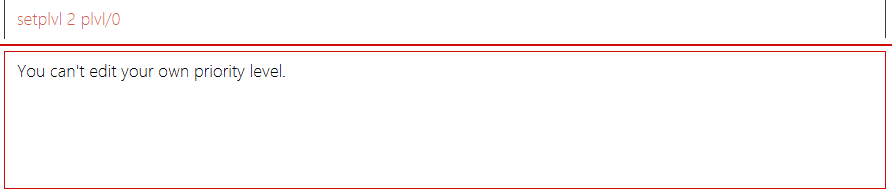
Appendix B: Privileges in accordance to priority level
Priority Level | Typical Roles | Privileges |
---|---|---|
BASIC (3) |
- Intern |
- Edit your own particulars |
MANAGER (2) |
- Head of Departments |
- All privileges of a BASIC Priority Level |
I.T. UNIT (1) |
- I.T. Department |
- All privileges of a MANAGER Priority level |
ADMINISTRATOR (0) |
- Branch Manager |
- All privileges of a MANAGER Priority level |
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Session component
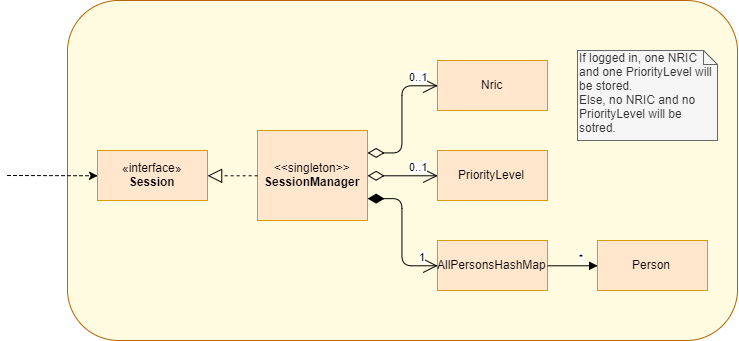
API : SessionManager.java
The Session
component,
-
stores the identity of the user who is logged in to the app during runtime.
-
provides the necessary APIs for functions that require elevated user status, to inquire from
SessionManager
as to whether the user is logged in, and also to inquire if the user has sufficient level of access to execute that particular function.
AllPersonsHashMap
is a hash map that stores all the persons from the AddressBook into the varialbe. This improves the efficiency
of logging in, and also for functions that require the details of the logged in person.
Priority level restriction feature
All employee accounts contain a priority level field. Depending on the priority level that they are given, administrative operations may be executed if they have sufficient priority level required to do so.
Implementation
Here is a code snippet of executing the AddCommand function. In this example, adding a person requires a person to be logged in with a priority level of ADMINISTRATOR or above, in order to execute this function:
@Override
public CommandResult execute(Model model, CommandHistory history) throws CommandException {
requireNonNull(model);
SessionManager sessionManager = SessionManager.getInstance(model);
/**
* Throws exception if user is not logged in.
*/
if (!sessionManager.isLoggedIn()) {
throw new CommandException(SessionManager.NOT_LOGGED_IN);
}
/**
* Throws exception if user does not have the required access level.
*/
if (!sessionManager.hasSufficientPriorityLevelForThisSession(PriorityLevelEnum.ADMINISTRATOR)) {
throw new CommandException(String.format(PriorityLevel.INSUFFICIENT_PRIORITY_LEVEL,
PriorityLevelEnum.ADMINISTRATOR));
}
// ... Complete the execution, now that user is verified to be an admin.
}
In the above code, adding a person requires the user that is logged in to the application to have priority level of ADMINISTRATOR. Hence, a method in SessionManager will be called to ascertain if the logged in user does indeed have sufficient priority level of an ADMINISTRATOR.
The following sequence diagram below shows the flow of how the feature works:
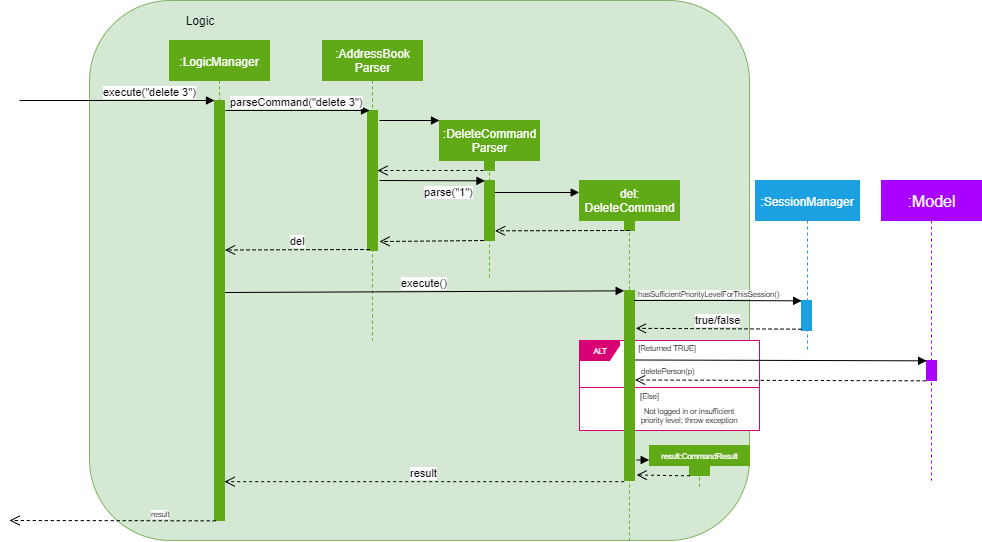
Design Considerations
Aspect: Implementation of elevated user rights
As this is a CLI-driven application, we prioritized on the speed and efficiency of getting things done over security, which will result in users having to execute multiple commands just to get an administrative command executed.
-
Alternative 1 (current choice): Able to perform administrative rights the moment a user with elevated priority level is logged in.
-
Pros: Able to immediately perform administrative functions the moment user is logged in, such as adding or deleting employees.
-
Cons: Potential security issue; user with admin rights may accidentally forget to logout, resulting in other people with ill intentions to execute administrative functions without the permission of the actual user.
-
-
Alternative 2: Users with elevated priority level will need to type in a command to enable "super user" status prior to executing any administrative functions.
-
Pros: Better security; company resources will be less-prone to abuse by employees with ill intentions.
-
Cons: Less efficient in getting things done. Reason being, this is a CLI-driven application. Hence, implementing this alternative means that users will need to key in multiple commands just to get administrative functions done as a trade-off to beefing up security.
-
Login to the application
-
Logs in to the application using a registered NRIC and associating password when not logged in prior to executing the command.
-
Prerequisites: -
-
Login NRIC has to be registered in the application, either through the sample data or added manually by an administrator.
-
You are not logged in prior to testing this function.
-
-
Test case:
login ic/S1234567E pwd/Password
Expected: Status message box states that you are logged in successfully, with your name, and your schedule (if any). Status bar will now show that you are logged in with the corresponding name of the user who logged in. -
Test case:
login ic/S12345678 pwd/Password
Expected: Not logged in. Format of NRIC is incorrect and Status message box shows the NRIC constraints. Status bar remains unchanged. -
Test case:
login ic/S1234567E pwd/high-five
Expected: Not logged in. Password format is incorrect and Status message box shows password constraints. Status bar remains unchanged. -
Test case:
login ic/S1234567E
Expected: Not logged in. Command format is invalid and Status message box shows the command usage. Status bar remains unchanged. -
Test case:
login ic/S1234567E pwd/SomeRandomPwd
Expected: Not logged in. Password does not match the one that’s registered with the app; Status message box shows error. Status bar remains unchanged.
-
-
Logs in to the application using a registered NRIC and associating password when already logged in prior to executing the command.
-
Prerequisites: You are already logged in prior to testing this function.
-
Test case:
login ic/T1234567E pwd/Password
Expected: Status message box shows the message: -
You are already logged in. Logout first before logging in again
.
Login status remain unchanged; status bar remain unchanged.
-
Checks the login status of the application
-
Checks the login status of this app. For this case, someone is logged in to the app.
-
Prerequisites: Someone is logged in to the app.
-
Test case:
checkloginstatus
Expected: Status message box shows the NRIC of the user who is logged in to the app.
-
-
Checks the login status of this app. For this case, user is not logged in prior to executing this command.
-
Prerequisites: User is not logged in prior to executing this command.
-
Test case:
checkloginstatus
Expected: Status message box shows that you are not logged in.
-
Logout of the application
-
Logs out of the application.
-
Prerequisites: User is logged into the app prior to executing this command.
-
Test case:
logout
Expected: Status message box shows that you have successfully logged out. Status bar footer now shows:Not logged in
.
-
Change priority level of employee
Refer to Appendix B of User Guide for the list of priority level. |
-
Change the priority level of an employee with one logged in as ADMINISTRATOR.
-
Prerequisites: -
-
You must be logged in as an administrator.
-
The following test cases assume that you are logged in as Alex Yeoh, an administrator with NRIC of
S1234567E
and password ofPassword
. Modify the test cases accordingly if you have made any modifications to the employee list prior to executing the following test cases.
-
-
Test case:
setplvl 1 plvl/3
Expected: Priority level of the employee at index 1 gets updated to a priority level of BASIC. Status message box shows that the priority level of the name of employee at index 1 has been changed to BASIC. -
Test case: Attempting to change your own priority level
setplvl 2 plvl/3
Expected: Priority level of the logged in user does not get changed. Status message box shows error that you are not allowed to update your own priority level. -
Test case:
setplvl 1 plvl/999
Expected: Priority level of the employee at index 1 is not updated. Input priority level is out of the range of valid priority level values. Status message bar shows error message.
-
-
Attempt to change the priority level of an employee with one logged in without admin rights.
-
Prerequisites: You must be logged in with an account that is not of ADMINISTRATOR priority level.
-
Test case:
setplvl 2 plvl/3
Expected: Priority level of the employee at index 2 is not updated. The user who is attempting to change the priority level does not have the rights to do so. Status message box shows an error message.
-
Reset the application
-
Resets the application, by deleting
data/AddressBook.xml
anddata/leave.xml
, provided that any of the files exist.-
Prerequisites: -
-
User must be logged in with a priority level of
I.T. UNIT
. -
In order for the test to be efficient, you may ensure that the files
data/AddressBook.xml
anddata/leave.xml
exist in the folderdata
.
-
-
Test case: type command
reset
Expected: Application will exit. The two files will be deleted upon application exit. When you manually re-open the app, a sample list of employee and leave will be shown.
-