Overview
Bank Address Book (BankAB) is a business process management and workflow application platform, whereby its users are all the employees in a banking environment. This platform supports the basic daily routine of each employee such as:
-
Checking working schedule
-
Applying for leave application
-
Checking in and out during working hours
The managers and administrators will have higher priority access level of BankAB such as changing the priority level of the employee and approve or reject leave requests.
Summary of contributions
-
Major enhancement: Added commands and enhancements related to
Department
andSort Command
.-
What it does: It provides the end user to be able to perform Department related functions such as list and filter departments, changing an employee’s department, and sorting the list based on employee’s name or department.
-
Justification: This feature caters to users who wants to have their employee’s list sorted, the ability to list out the available departments, filtering out the employee’s list based on their departments and changing an employee’s department.
-
Highlights: This enhancement enhances the user experience to use the commands that are related to
Department
. It required an understanding on the user profile in order to create the commands that will benefit the users. The implementation is challenging as it requires an understanding on the regular expressions in Java to allow the commands to work as how it is and to minimise bugs from happening due to incorrect regular expressions.
-
-
Code contributed: RepoSense Dashboard
-
Other contributions:
-
Project management:
-
Managed releases
v1.1
-v1.4
(5 releases) on GitHub.-
Managed the release of .jar files and the descriptions of the release.
-
-
Setting up of issue tracker, milestones and tags.
-
Managed vetting through and approving of pull requests.
-
Ensures the team are able to complete the requirements for every milestones.
-
-
Enhancements to existing features:
-
Made modifications to the existing implementation after the feedback received from the first practical exam: #129
-
-
Documentation:
-
Community:
-
Tools:
-
Integrated GitHub plugins (Travis-CI, AppVeyor, Coveralls and Codacy) to the team repository
-
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Change the department of an employee : setdepartment
|
Changes the department of an employee.
Format: setdepartment INDEX d/DEPARTMENT
Alias: sd
Example: setdepartment 3 d/Junior Management
Changes the employee department with ID 3 in the address book to Junior Management
.
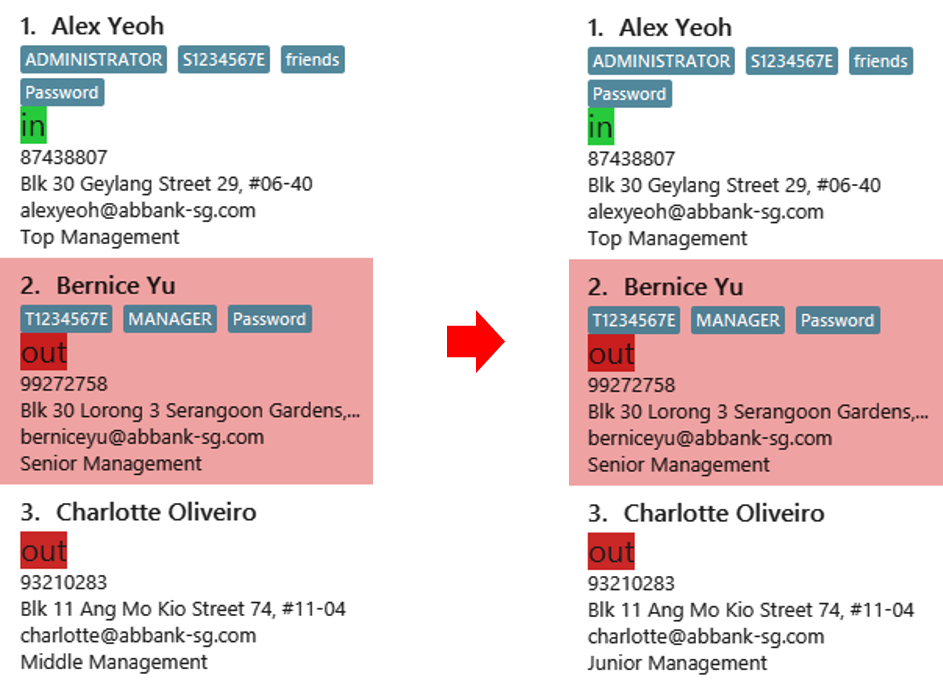
Sort address book contacts: sort
|
Sorts the employees or departments of the current list in sorted order.
The list can be sorted in ascending or descending order.
Format: sort FIELD ORDER
This operation only supports the following fields and orders
|
Examples:
-
sort name asc
Sorts the employee names in the list in ascending order
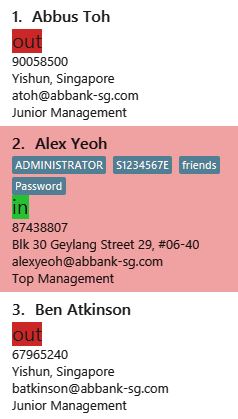
-
sort department desc
Sorts the department names in the list in descending order
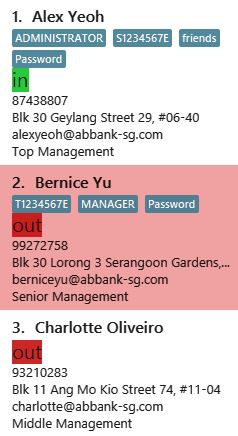
List department : listdepartment
|
Shows a list of departments available in the addressbook.
Format: listdepartment
Alias: ld
Example: listdepartment
Returns a list of departments available in the addressbook currently. Listing of department names are in ascending order.

Filtering department : filterdepartment
|
Filter departments and list out the employees who are in the department.
Format: filterdepartment KEYWORD [MORE KEYWORDS]
Alias: fd
-
The search is case insensitive. e.g.
junior
will match employees inJunior Management
-
Only full words will be matched. e.g.
junio
will not matchJunior Management
-
Filtering of more than one department will list out the employees in the departments.
Examples:
-
filterdepartment junior
Returns a list of employees who are in Junior Management
.
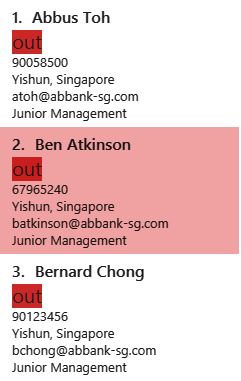
-
filterdepartment junior senior
Returns a list of employees who are in Junior Management
and Senior Management
.
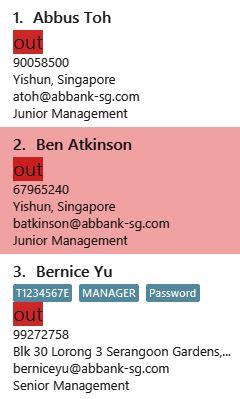
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
FilterDepartment feature
Implementation
The filterdepartment
is a command that filters out department to list a particular department employee’s list.
The filterdepartment
mechanism is facilitated by the FilterDepartmentCommandParser
, which parses input arguments and creates a new FilterDepartmentCommand
object.
The FilterDepartmentCommandParser
, which implements the Parser
interface, parses the arguments inputted in to the CLI, and checks if the user input conforms the expected format.
Code snippet from FilterDepartmentCommandParser.java
that shows the parsing of arguments and checking for invalid arguments:
public FilterDepartmentCommand parse(String args) throws ParseException {
String trimmedArgs = args.trim();
trimmedArgs = trimmedArgs.toLowerCase();
if (trimmedArgs.isEmpty() || trimmedArgs.contains("management")) {
throw new ParseException(
String.format(MESSAGE_INVALID_COMMAND_FORMAT, FilterDepartmentCommand.MESSAGE_USAGE));
}
...
...
}
In the second phase, the command is being executed in FilterDepartmentCommand
. The updateFilteredPersonList updates the filteredPersonList with the departments that matches the keywords use as the argument in FilterDepartmentCommand
.
The result of FilterDepartmentCommand
execution is encapsulated as a CommandResult
object and returns it to the LogicManager
and subsequently to the UI and display the filtered department employee’s list.
Code snippet from FilterDepartmentCommand.java
:
public class FilterDepartmentCommand extends Command {
...
...
private final DepartmentContainsKeywordsPredicate predicate;
public FilterDepartmentCommand(DepartmentContainsKeywordsPredicate predicate) {
this.predicate = predicate;
}
@Override
public CommandResult execute(Model model, CommandHistory history) {
requireNonNull(model);
model.updateFilteredPersonList(predicate);
return new CommandResult(
String.format(Messages.MESSAGE_PERSONS_LISTED_OVERVIEW, model.getFilteredPersonList().size()));
}
...
...
}
The sequence diagram below demonstrates the interaction within the Logic
component of FilterDepartmentCommand
:
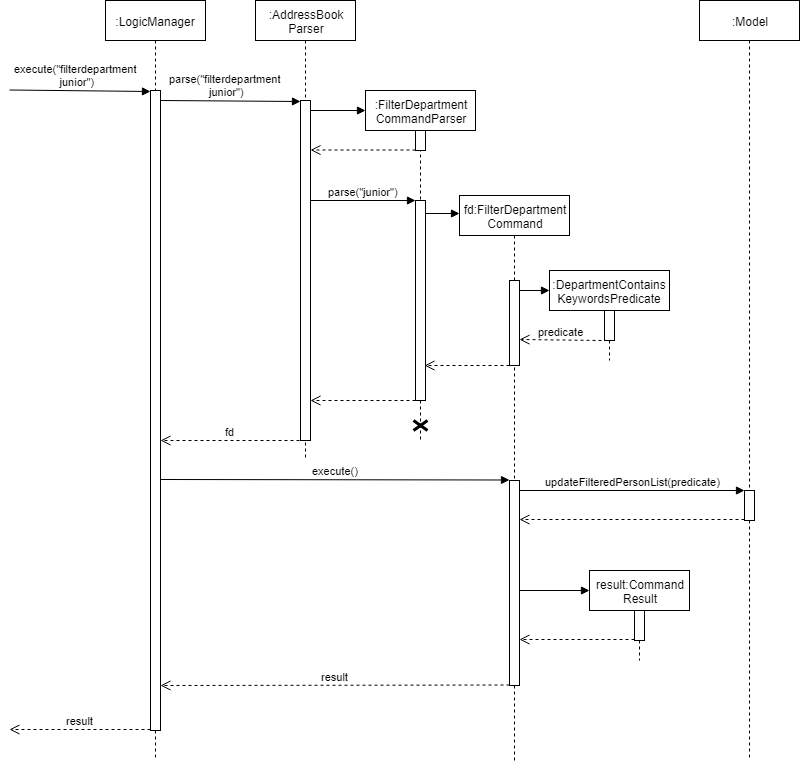
Design Considerations
Aspect: Implementation of FilterDepartment
command
-
Alternative 1 (current choice): A separate command for filter department.
-
Pros: Command works more accurately and efficiently as it focuses on the keywords related to
Department
. -
Cons: The implementation of another command may be unnecessary as we can integrate
find
command to find for departments.
-
-
Alternative 2: Using
find
command to filter department name.-
Pros: One command works for finding name and to filter department names.
-
Cons: The command may not work properly. For example, there is an employee whose name is
Junior
fromSenior Management
. But if we only want to filter employees who are inJunior Management
, the command will output people who are inJunior Management
and the employeeJunior
.
-